How to round to 2 decimal places in JavaScript?
When it comes to rounding a number to two decimal places in JavaScript, a developer has a lot of options. It can be done with:
The toFixed() function.
The Math.round() function.
A helper function.
- The lodash round() function.
In this article, I will explain, in detail, how to use each one of them, as well as show real-life examples for better understanding.
Let's get to it 😎.
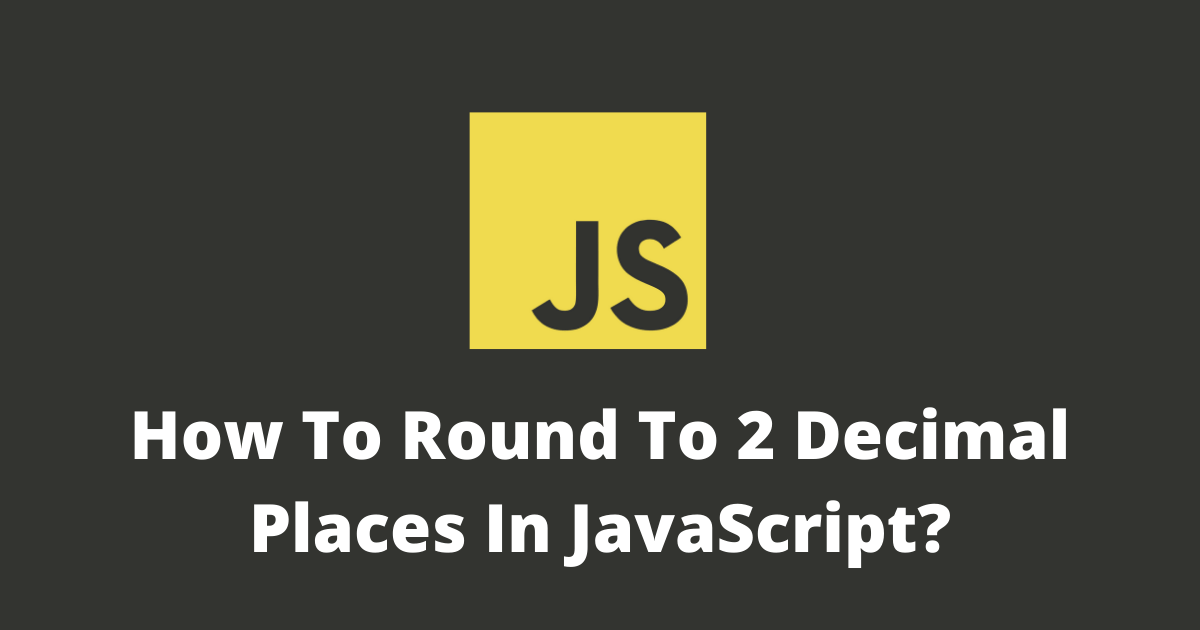
How to round a number using the toFixed() function?
The first and easiest solution is to use the toFixed() function.
typescriptconst number = 18.154;
// The output of this is: 18.15
console.log(parseFloat(number.toFixed(2)));
This method is the easiest but not the most accurate one.
For example: for 4.005 it will give 4.
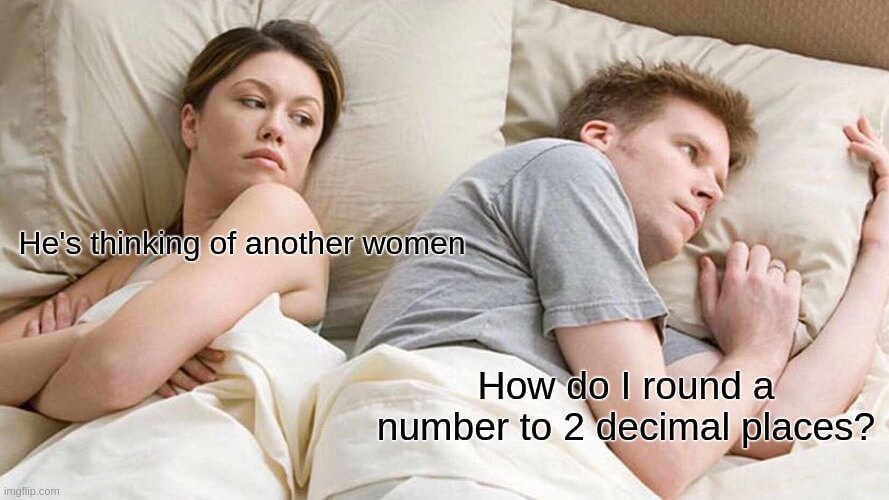
How to round a number using the Math.round() function?
You can round a number to 2 decimal places by using the Math.round() function.
typescriptconst number = 18.154;
// The output of this is: 18.15
console.log(Math.round((number + Number.EPSILON) * 100) / 100);
How to round a number using a custom helper function?
You can also round a number by creating a helper function that will use the Math.round() function and some JavaScript magic.
typescriptconst number = 18.154;
function roundTo(n, place) {
return +(Math.round(n + "e+" + place) + "e-" + place);
}
// The output of this is: 18.15
console.log(roundTo(number, 2));
How to round a number with lodash?
Rounding a number can be hard to do by yourself because you need to think about a lot of use cases.
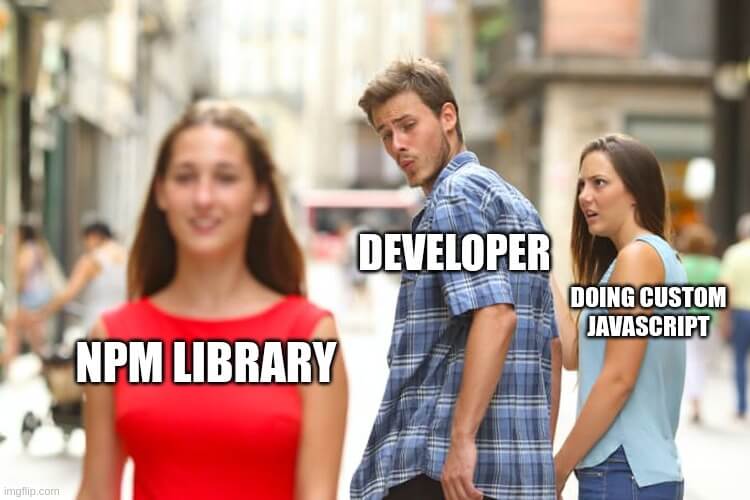
That's why you can install a library that will do it for you. I like to use the lodash library, which is a library that contains a lot of utility functions to use.
Here is a how to round a number with lodash:
javascriptimport { round } from 'lodash';
// The output of this is: 18.15
console.log(round(18.154, 2));
Final Thoughts
As you can see, you have a lot of options to round a number to a specific decimal place in JavaScript.
Which to choose is a matter of preference. For my part, most of the time, I use a helper function method that I create in my utils and import when I need to.
Finally, here are some of my other related JavaScript tutorials: